- Pokémon Essentials Version
- v21.1 ✅
- Also compatible with
- v21
This script is designed to implement and manage a dynamic darkness effect in the game, adding a semi-transparent black layer that can vary in opacity and adapt to different scenarios. It is useful for creating immersive atmospheres, such as nighttime environments, shadowy dungeons, weather events, or any scene requiring lighting adjustments.
SCRIPT:
Use example based on conditionals (game variable, or internal ruby variables, same works)
The system requires parallel process event for persistent darkness level data.
On exit of map, will dispose the Darkness Effect:
Main Features
- Darkness Creation:
- Generates a dark layer using a Sprite that covers the entire screen.
- The layer has a high depth (z) to ensure it always appears above other graphical elements.
- Opacity Control:
- Allows direct adjustment of the darkness intensity with a numeric value (opacity) ranging from 0 (fully transparent) to 255 (fully opaque).
- Smooth Transitions:
- Includes a function to perform gradual opacity transitions (fade_to), useful for effects like progressive darkening or gradual brightening during specific scenes.
- Management and Reuse:
- Provides global methods to initialize, adjust, fade, and release the darkness layer when no longer needed.
- A function ensures the effect is properly initialized (ensureDarkness), avoiding errors during gameplay.
- Optimization:
- Resources associated with the effect are properly released using the dispose method, helping prevent performance issues.
Expected Usage
- Atmosphere: Dynamically change the intensity of darkness to reflect ambient lighting changes.
- Narrative Scenes: Use opacity transitions to add drama to key events in the game.
- Gameplay: Integrate the effect into mechanics, such as reducing visibility during specific events or in areas where lighting is part of the challenge.
Global Methods
- setDarknessIntensity(value): Instantly adjusts the opacity of the darkness.
- DarkenessIntensityFade(value_one, value_two): Gradually changes the opacity from the current level to value_one over value_two frames.
- disposeDarkness: Releases resources associated with the darkness effect.
- recallDarkness: Reinitializes the darkness effect if it needs to be reused.
- ensureDarkness: Verifies and initializes the darkness layer if it doesn’t already exist.
SCRIPT:
Ruby:
# Clase para manejar el efecto de oscuridad en el juego
class DarknessEffect
attr_reader :sprite
def initialize
@sprite = Sprite.new
@sprite.z = 9999 # Asegura que esté por encima de otros elementos
end
def create_darkness_bitmap
@sprite.bitmap = Bitmap.new(Graphics.width, Graphics.height)
@sprite.bitmap.fill_rect(0, 0, Graphics.width, Graphics.height, Color.new(0, 0, 0))
end
# Getter y setter para la opacidad
def opacity=(value)
@sprite.opacity = value
end
def opacity
@sprite.opacity
end
# Cambia gradualmente la opacidad
def fade_to(target_opacity, duration = 60)
start_opacity = @sprite.opacity
duration.times do |i|
@sprite.opacity = start_opacity + (target_opacity - start_opacity) * i / duration
Graphics.update
end
end
def dispose
@sprite.dispose if @sprite
end
def ensure_darkness_initialized
# Check if the sprite doesn't exist or has been disposed
if @sprite.nil? || @sprite.disposed?
create_darkness_bitmap
return true
end
false
end
end
# Inicialización global del efecto de oscuridad
$game_darkness_effect ||= DarknessEffect.new
#Set Darkness value immediately
def setDarknessIntensity(value)
$game_darkness_effect.opacity = value
end
#Darkness value_one, applies with fade effect of value_two frames.
def DarkenessIntensityFade(value_one, value_two)
$game_darkness_effect.fade_to(value_one, value_two)
end
#Dispose all darkness system
def disposeDarkness
$game_darkness_effect.dispose
end
#Reinitialize Darkness System
def recallDarkness
$game_darkness_effect.initialize
$game_darkness_effect.create_darkness_bitmap
end
#Checks if Darkness System is complete
def ensureDarkness
$game_darkness_effect.ensure_darkness_initialized
end
#Recreates only the darkeness bitmap.
def recallDarkSprite
$game_darkness_effect.create_darkness_bitmap
end
Use example based on conditionals (game variable, or internal ruby variables, same works)
The system requires parallel process event for persistent darkness level data.
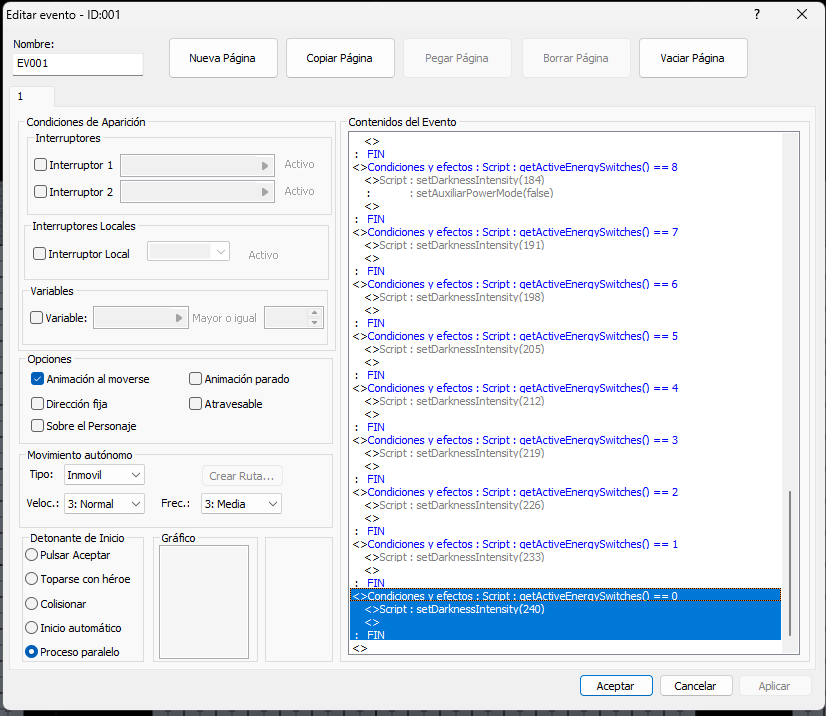
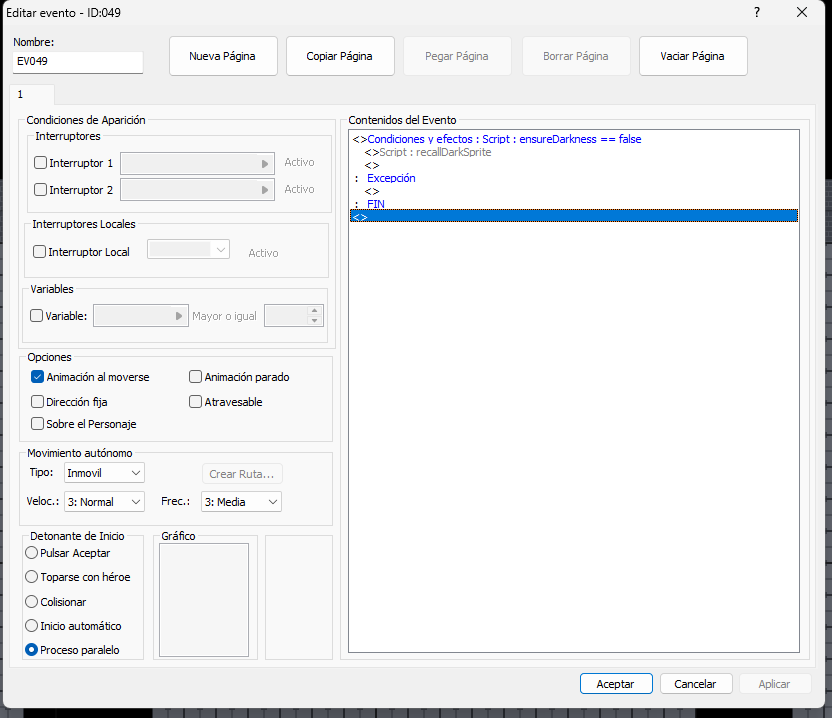
On exit of map, will dispose the Darkness Effect:

- Credits
- IgnathiusNZX